Launching Firefox, IE and Chrome Browsers in Selenium WebDriver
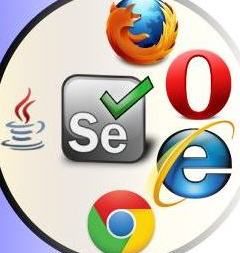
As you know, Selenium supports different browsers including Firefox, Chrome, IE, Safari, etc. To automate test cases, of a web application, the first thing you need to do is to open or launch a browser and then navigate to the web page you want to automate. In this article, we will be seeing how to launch the various browsers supported by Selenium.
To execute scripts on different browsers, we need to download their corresponding drivers which acts as standalone servers to execute your script on the required browser. These driver servers acts as a link between your tests in Selenium and the browser.Remember Selenium WebDriver is an Interface. A class that implements the interface agrees to implement all of the methods defined in the interface. So, the corresponding drivers of each browsers are the classes which implements the WebDriver interface.
Now, let us see how we can launch the Firefox, IE and Chrome browsers. You need to download the following drivers to work with different browsers.
- Firefox- Mozilla GeckoDriver
- IE- Internet Explorer Driver
- Chrome- ChromeDriver
Mozilla Firefox
Up to Selenium 2.53 versions, Firefox was the native browser for Selenium WebDriver and the user did not have to download any additional package or driver executable for launching Firefox browser. But, from Selenium 3.0, you need to download the Gecko driver which will interact with the Firefox browser. You can download the driver from https://github.com/mozilla/geckodriver/
Now, to launch the browser you need to write code for the following steps:
1.Set the system property path to the location of Geckodriver executable.
To set path, the command is:
System.setProperty("webdriver.gecko.driver"," Path of gecko driver exe file ")
2.Create a new instance for the FirefoxDriver.
3.Now, you have successfully launched the browser and can navigate to the web page.
Here is the code to launch the facebook home page in Firefox browser and print the page title in the output console.
import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.firefox.FirefoxDriver; public class fireFoxDriver { public static void main(String[] args) { System.setProperty("webdriver.gecko.driver"," D:\\Selenium_files\\geckodriver-v0.14.0-win64\\geckodriver.exe"); //Sets the system property path WebDriver driver= new FirefoxDriver(); //Create new instance for FirefoxDriver driver.get("http://www.facebook.com"); //Navigate to Facebook home page System.out.println(driver.getTitle());//Prints the Page Title driver.close(); //Closes the browser } }
Internet Explorer
To launch the IE browser , you need to download the IEDriver server executable file which will link your tests in Selenium and the IE browser. You can download the driver from https://www.seleniumhq.org/download/ . 32 bit and 64 bit compatible versions are available. Download the one depending on your system configuration.
The steps to launch are similar to what you did for Firefox.
1.Set the system property path to the location of InternetExplorerDriver executable.
To set path, the command is:
System.setProperty("webdriver.ie.driver"," Path of IE driver exe file ")
2.Create a new instance for the InternetExplorerDriver.
3.Now, you have successfully launched the browser and can navigate to the web page.
Here is the code to launch the facebook home page in Chrome browser and print the page title in the output console.
import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.ie.InternetExplorerDriver; public class IEDriver { public static void main(String[] args) { System.setProperty("webdriver.ie.driver","D:\\Selenium_files\\IEDriverServer_x64_3.0.0\\IEDriverServer.exe"); //Sets the system property path WebDriver driver= new InternetExplorerDriver(); //Create new instance for IEDriver driver.get("http://www.facebook.com"); //Launch Facebook home page System.out.println(driver.getTitle()); //Prints the page title. driver.close(); //Close the browser } }
Google Chrome
To launch the chrome browser you, you need to download the chromeDriver executable file which will link your tests in Selenium and the Chrome browser. You can download the driver from https://sites.google.com/a/chromium.org/chromedriver/ . The steps to launch chrome browser are similar to what you did for Firefox/IE.
1.Set the system property path to the location of ChromeDriver executable.
To set path, the command is:
System.setProperty("webdriver.chrome.driver"," Path of chrome driver exe file ")
2.Create a new instance for the ChromeDriver.
3.Now, you have successfully launched the browser and can navigate to the web page.
Here is the code to launch the facebook home page in Chrome browser and print the page title in the output console.
import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; public class ChromeDriver { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver","D:\\Selenium_files\\chromedriver_win32\\chromedriver.exe"); //Sets the system property path WebDriver driver= new ChromeDriver(); //Create new instance for ChromeDriver driver.get("http://www.facebook.com"); //Launch Facebook home page System.out.println(driver.getTitle()); //Prints the page title. driver.close(); //Close the browser } }
Hurray! Now, you know how to launch these browsers using WebDriver, next steps in writing Selenium scripts are identifying the webElements on your web page and performing operations on them. Read on our article on Selenium WebDriver commands and Finding WebElement locators to move on! Enjoy scripting!
How much is a great User Experience worth to you?
Browsee helps you understand your user's behaviour on your site. It's the next best thing to talking to them.
