Selenium WebDriver Commands
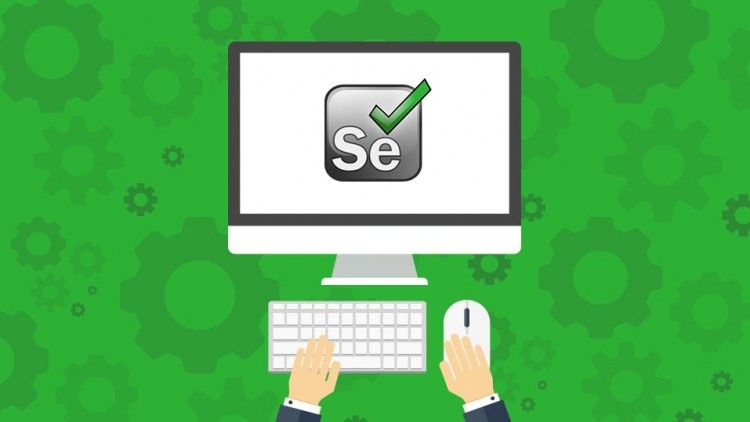
In Selenium WebDriver, to automate test cases, we need to identify the web element for the step and perform some actions on the web element. The web element may be any object on the web page like button, link, drop down, checkbox, radio button, image, textbox, some text on the page, etc. In Selenium, these Web elements are identified using ‘Locators’ and the Selenium WebDriver implementation uses Selenium commands or methods to perform actions on these web elements. A method can be considered to be a collection of statements grouped together to perform an action.
Using these methods/commands, all types of actions that we typically perform on a website can be done like click, scroll, select, de-select, mouse hover, etc. To put it simple, automation scripts written in Selenium use the selenium WebDriver methods for emulating the user actions on a web page. So, basically the element locators and WebDriver methods comprise the main elements of your test case.
Now that we have seen what is WebDriver methods, let’s see how can we invoke these methods in Selenium WebDriver.
To understand this, first thing you need to note is that Selenium WebDriver is an interface which consists of abstract methods. So, the first step in creating a selenium script is to create a WebDriver Instance. Once you create an instance, you can use this instance to invoke methods. This can be done by assigning the instance to a variable, which can be used to invoke the methods. Here we create a WebDriver instance and assign it to the ‘driver’ object.
Now, we can use this driver object to invoke all the methods available in the Selenium WebDriver interface. Now, if you type in driver and press dot(.) key, all the methods of WebDriver will be listed as shown below.
As you see, there are many methods available. Covering all the methods may be beyond the scope of this article. But we will try to cover all major commands which you would need to develop automation scripts. When you learn something, it’s always better to classify things and learn each group one by one. So for better understanding, we can classify the Selenium WebDriver commands into following three categories and take them one by one.
- Browser related methods
- Navigation methods
- WebElement methods
Browser related Methods
These methods are used to control the browser.
Get Command
This method is used to launch a web page. The input string is the URL of the page to be launched . This method does not return anything.
Command – driver.get(“url”);
Get Title Command
This method is used to get the title of the current page. There is no input parameter required and it returns the Title of page as a string value. Hence, the output of this command must be stored in a String object or variable.
Command – driver.getTitle();
Get Current URL Command
This method is used to get the URL f the current page. There is no input parameter required and it returns the URL of current page as a string value. Hence, the output of this command must be stored in a String object or variable.
Command – driver.getCurrentUrl();
Get PageSource command
This method is used to get the source code of the current page. This method too doesn't not take any input parameter and returns the entire page source of the current webpage as a string value.
Command- driver.getPageSource();
Close command
This method is used to close the current window that Selenium WebDriver is working on. This method does not accept any input parameter and returns nothing.
Command- driver.close();
Quit Command
This method is used to close all windows opened. This method too does not accept any input parameter and returns nothing.
Command- driver.quit();
Navigation Methods
These methods are used to navigate from one page to another in the browser.
Navigate To Command
It does the same thing as driver.get(“url”) and navigates to the URL mentioned in the command. It takes the URL of page as input parameter and returns nothing.
Command- driver.navigate().to(“url”);
Forward Command
It does the same operation as clicking the forward button in your browser. It does not accept any input parameter and returns nothing.
Command – driver.navigate().forward();
Back Command
This method is used to perform same action as clicking the ‘back’ button in the browser. This command too neither accepts nor returns anything.
Command- driver.navigate().back();
Refresh Command
Method used to refresh the current page. This command too neither accepts nor returns anything.
Command- driver.navigate().refresh();
WebElement Methods
These methods are used to do various actions using the web elements on the web page.
FindElement Command
This method is used to select or find a web element on the web page. The web element is identified using Locators. So, in order to locate a web element we use the below line of code.
WebElement element= driver.findElement(By.locator(“locator value”));
The locator may be anything like id, name, LinkText, Xpath, CSS ,PartialLinkText, ClassName or TagName. Once you type in By followed by dot (.), you get the list of all locator strategies available in WebDriver as shown below.
The findElement method returns an object of type WebElement. Once you identify an element you can perform different actions on the element. Now, if you type in dot(.) following your findElement statement, Eclipse will give you the list of actions you can perform on the web elements as shown below.
######**FindElements Command** FindElements method is also used to identify WebElements in same way as the FindElement method. The difference between two is that the the FindElement returns a single WebElement while the FindElements method returns a list of WebElements. So, if there are multiple WebElements having the same locator value, the FindElement command returns the first WebElement with matching locator while FindElements returns all the WebElements with matching locator as a list of WebElements. `Command- driver.findElements(By.locator(“locator value”));`
Clear Command
This command is used to clear the values of any text entry element.
Command- element.clear();
SendKeys Command
This command is used to enter some text into a web element like an input box/ text area and set its value. It does same as typing in from a keyboard the char sequence given in the command.
Command- element.sendkeys(“text”);
Click command
This command is used to click on any web element. It may be button, link, text box, radio button, check box or any such web element on a web page. The command neither accepts nor returns anything.
Command- element.click();
Submit command
If the web element is the submit button on a form, this command works better compared to click command although the action performed is same. This is because if the click causes a new page to be loaded, this method will wait until the new page gets loaded.
Command- element.submit();
IsSelected command
This command is used to determine whether an element is selected or not. This can be used for web elements like checkboxes, radio buttons or select options. The command returns a Boolean value true if element is selected or checked, otherwise returns false. The return variable type is Boolean(true/false)
Command- boolean status= element.isSelected();
IsDisplayed command
This command is used to determine whether an element is displayed on the web page. It returns a bollean value(true/false). The command returns true if the element is displayed on the page, otherwise it returns false.
Command- boolean status= element.isDisplayed;
IsEnabled command
This command is used to determine is an elements is enabled or not. Sometimes, you would have to test for scenarios where some area on the web page has to be disabled before some action. For eg- the Login button should be enabled only after the username and password fields are entered. This command can be used to test such scenarios.
Command- boolean status= element.isEnabled();
getTagName Command
This method is used to get the tag name of a web element. It returns the TagName as a string value. Note that it just returns the TagName and not the value for the attribute.
For eg: <input type= “email”>
For the above line of code, it just reurns input and not email.
Command- String tagName= element.getTagName();
getCSSValue command
This command can be used to get the CSS property of any given element. It returns a string value.
Command- String CSSValue= element.getCSSValue();
getAttribute Command
This command is used to get the value of any attribute of a given element. The attribute may be id, name, class, etc. The command accepts the attribute required as a string value and returns its value also as a string.
Command- String attValue= element.getAttribute(“attribute name”);
getSize command
Used to get the dimensions of a web element. It returns the dimension object.
Command- Dimension dimension= element.getSize();
getText command
This method is used to get the inner text of any web element. It returns a string value.
Command- String text= element.getText();
getLocation command
This is used to get the co-ordinates of an element on a web page. It returns the Point object, which gives the x and y co-ordinates of the given element.
Command- Point point= element.getLocation();
The article does not cover all the WebDriver command/methods available. But we have tried to give an overview of most of the commands you would need to use frequently for creating automation scripts using Selenium. Once you get into scripting, you will learn by yourself to use other methods whenever necessary. This is good enough to start with!
How much is a great User Experience worth to you?
Browsee helps you understand your user's behaviour on your site. It's the next best thing to talking to them.
