Browser Storage: A Comparative Analysis of IndexDB, Local Storage, and Session Storage
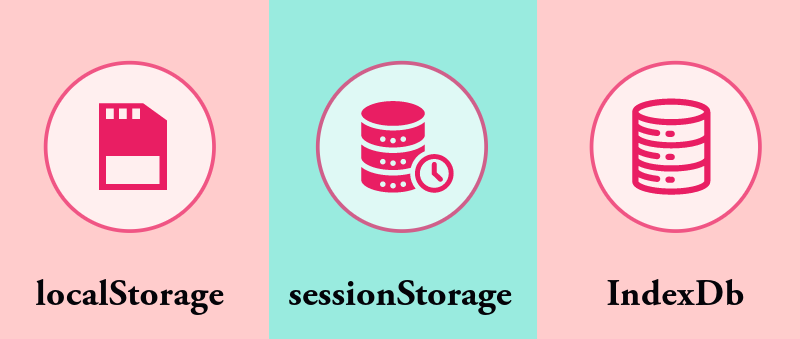
Client side storage is a key use case for web developers and any new interface is usually welcome by them. Local Storage, and Session Storage have been extensively used in the last few years, so today we will learn how to use IndexDb.
We dive into each storage mechanism's unique features, use cases, and benefits. Whether you're a developer seeking to optimise data handling or an enthusiast eager to explore the inner workings of web storage, this article is for you.
IndexDB: The Robust and Persistent Storage Solution

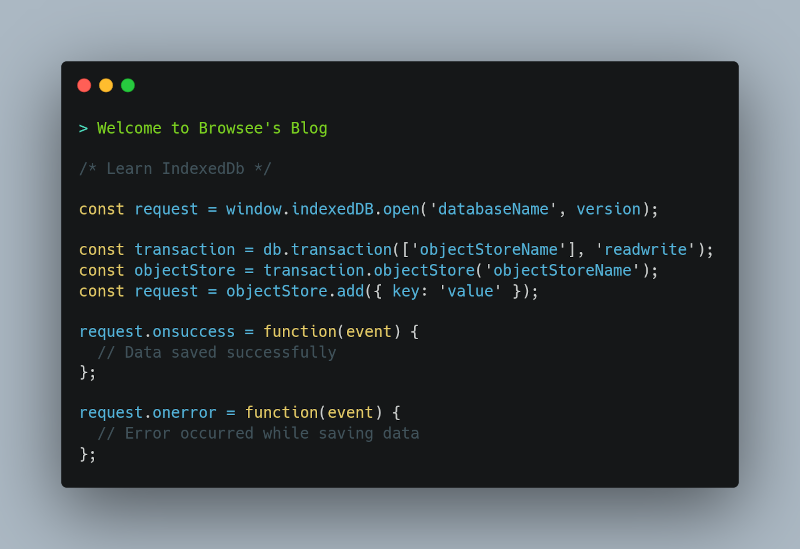
IndexDB is a powerful client-side storage mechanism that allows developers to store significant amounts of structured data. IndexedDB is a game-changer in web development, offering a robust data storage solution.
With seamless data-saving and retrieval capabilities, developers can create efficient web applications with offline functionality and enhanced user experiences. You can unlock a world of possibilities by harnessing the unique features of IndexedDB and optimising data management techniques.
Key features of IndexDb
Asynchronous API: Supports efficient data handling without blocking the main thread.
Key-Value Storage: Stores data in key-value pairs, providing fast retrieval and indexing capabilities.
Indexed Queries: Enables complex querying using indexes, improving search performance.
Transactions and Object Stores: Facilitates atomic operations and data separation using object stores.
Persistence: Offers long-term data storage, surviving browser restarts and system crashes.
Use Cases
Offline Web Applications: IndexDB allows applications to work seamlessly without an internet connection, syncing data upon reconnection.
Caching: Storing frequently accessed data locally reduces server load and enhances application responsiveness.
Data-Intensive Applications: Complex applications dealing with large datasets benefit from IndexDB's efficient indexing and querying capabilities.
Advantages Over Other Storage Mechanisms
High storage capacity: IndexDB can store large amounts of data, ranging from a few megabytes to gigabytes.
Complex data queries: Developers can perform advanced queries using indexes, enhancing application performance.
Durability: Data stored in IndexDB persists even when the browser is closed, or the system crashes.
Scalability: IndexDB scales well with large datasets, making it suitable for data-intensive applications.
Local Storage: Storing for the Long Haul
let person = { name: "John", age: 22 }; localStorage.setItem("profile", person); console.log(localStorage.getItem("profile"));

Local Storage is a simple key-value storage mechanism designed for persisting data on the client side. Local Storage operates within the web browser, allowing developers to store and retrieve data using simple key-value pairs.
It offers a straightforward API, with methods like setItem()
and getItem()
, making storing and retrieving data easy. Modern browsers widely support local storage, ensuring compatibility across different platforms and devices.
With Local Storage, developers can persist user preferences, form data, and other small-scale data, providing a seamless browsing experience for users.
Key Features
Easy-to-use API: Simple methods like setItem()
and getItem()
make data storage and retrieval effortless.
Synchronous Operations: Local Storage operates synchronously, blocking the main thread during read and write operations.
String-Based Storage: Stores data as strings, requiring serialisation and deserialisation for complex objects.
Limited Storage Capacity: Typically, local storage allows around 5-10MB of data storage.
Use Cases
User Preferences and Settings: Local Storage is commonly used to store user preferences, such as theme selection or language settings.
Form Data Persistence: Saving form data locally prevents data loss in case of accidental page refresh or browser closure.
Simple Data Caching: Storing small amounts of frequently accessed data to improve application responsiveness.
Advantages
Simplicity: Local Storage provides a straightforward API for storing and retrieving data with minimal effort.
Cross-Browser Compatibility: Modern browsers widely support local storage, ensuring broad compatibility.
Persistence: Data stored in Local Storage persists even after the browser or system restarts.
Lightweight: Local Storage is ideal for small amounts of data that do not require complex querying or indexing.
Session Storage: Lightweight and Transient Storage
const person = {'name': 'John Snow', knows: 'nothing'} sessionStorage.setItem('scores', JSON.stringify(person)); /** Gone as soon as tab is closed */
Session Storage is similar to Local Storage but designed for temporary data storage during browsing.
Session Storage is commonly employed in applications requiring transient states, such as shopping carts or multi-step forms. It provides a lightweight and efficient solution for storing and accessing temporary data during a user's browsing session.
By leveraging Session Storage, developers can ensure data persistence within a single tab while preserving user privacy by automatically clearing the data when the session ends.
Key Features
Same API as Local Storage: Session Storage shares the same API and capabilities as Local Storage.
Tab-Specific Storage: Data stored in Session Storage is isolated to a particular browser tab and is cleared when the tab is closed.
Size Limitations: Typically, Session Storage offers a similar storage capacity as Local Storage, around 5-10MB.
Use Cases
Shopping Carts: Session Storage is useful for storing temporary shopping cart data during a user's browsing session.
Multi-Step Forms: Storing form data across multiple steps allows users to resume their progress during a session.
Transient Application State: Temporarily storing application state data, such as active tabs or selected options.
Advantages
Isolation: Session Storage provides tab-specific storage, ensuring data separation and privacy.
Transience: Data stored in Session Storage automatically clears when the user closes the tab or browser, enhancing privacy and security.
Lightweight: Session Storage is suitable for small, transient data that does not require long-term persistence.
Comparison: IndexDB vs Local Storage vs Session Storage
In this section, we will compare the three storage mechanisms based on key parameters:
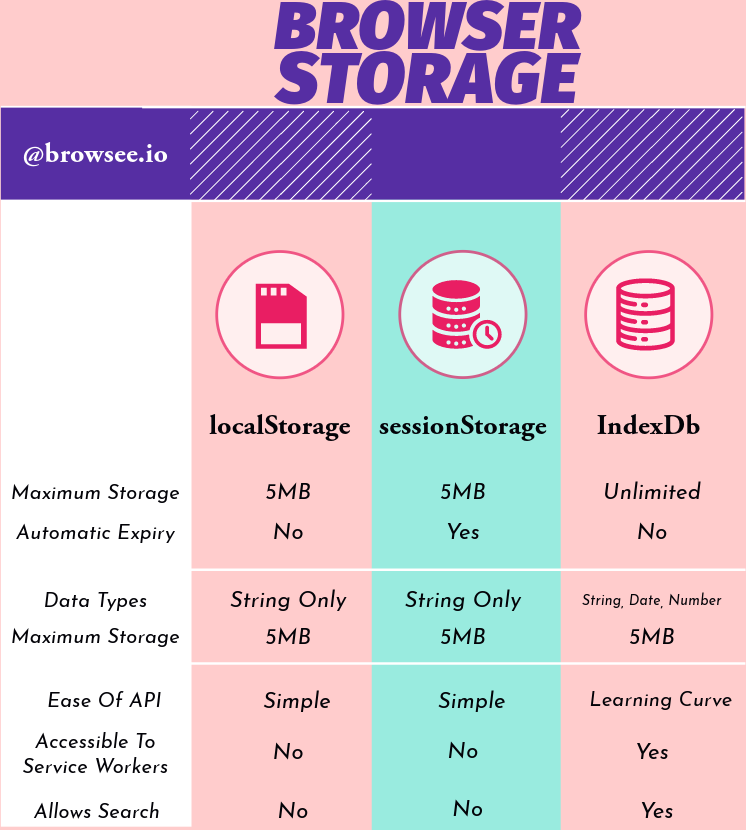
Performance
IndexDB: Offers asynchronous operations, enabling non-blocking data handling and improved application responsiveness. In general its slower in comparison to localStorage [1].
Local Storage: Synchronous operations may block the main thread during read and write operations, but is usually fast.
Session Storage: Similar to Local Storage.
Capacity and Limitations
IndexDB: Provides substantial storage capacity, ranging from megabytes to gigabytes, making it suitable for large datasets.
Local Storage: Typically allows 5-10MB of storage, limiting its use from small to medium-sized data sets.
Session Storage: Offers similar storage capacity as Local Storage, restricting its use to small-scale data storage.
Persistence
IndexDB: Data stored in IndexDB persists across browser restarts and system crashes, ensuring long-term durability.
Local Storage: Data remains persistent, surviving browser restarts and system crashes.
Session Storage: Data stored in Session Storage is temporary and clears when the user closes the tab or browser.
API
IndexDB: API is not intuitive and there is a learning curve involved. However, its the only option avaialble for storage when using service workers as localStorage is inaccessible to service workers. Also it allows a search by keyRange
which helps in partial string matching.
Example: you can can save a city list in the browser in IndexDb and during city selection power suggestions based on first few letters. This would save a roundtrips of city data from the server in case its a recurring use case.
Local Storage: Data remains persistent, surviving browser restarts and system crashes.
Session Storage: Data stored in Session Storage is temporary and clears when the user closes the tab or browser.
When comparing IndexDB, Local Storage, and Session Storage, it is evident that each has its own strengths and best use cases.
However, if we consider the overall performance, capacity, and durability, IndexDB emerges as the most potent and versatile storage mechanism. With its asynchronous operations, IndexDB ensures non-blocking data handling, resulting in improved application responsiveness.
Its substantial storage capacity ranging from megabytes to gigabytes, makes it suitable for managing large datasets effectively. Additionally, the persistence of data stored in IndexDB across browser restarts and system crashes ensures long-term durability, making it a reliable choice for critical applications.
Local Storage and Session Storage, while having their own merits, have some limitations regarding performance and storage capacity. Local Storage is better suited for smaller-scale data storage and more straightforward use cases, such as storing user preferences or form data. Session Storage, on the other hand, excels in handling transient data that needs to be cleared at the end of a browsing session.
In a Nutshell
IndexDB, Local Storage, and Session Storage are client-side storage mechanisms with distinct features and use cases.
IndexDB is ideal for handling large datasets and offline web applications, offering advanced querying and long-term persistence.
Local Storage is a simple key-value storage option for smaller-scale data with cross-browser compatibility.
Session Storage is suitable for temporary data storage during browsing and clearing data when the tab or browser is closed.
While each storage mechanism has its strengths, understanding its capabilities allows developers to choose the most suitable option based on the specific needs of their applications.
How much is a great User Experience worth to you?
Browsee helps you understand your user's behaviour on your site. It's the next best thing to talking to them.
