How to Write an Automated Test for Your Website Using Puppeteer?
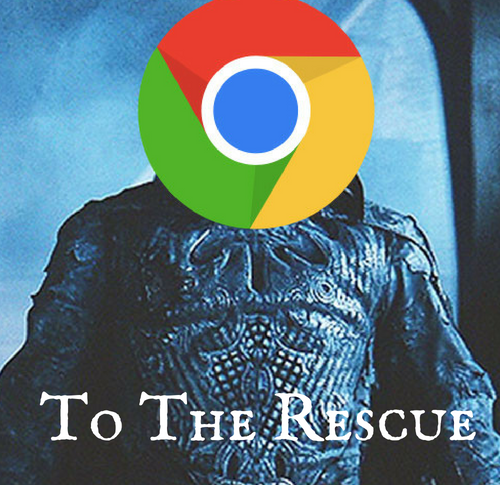
Chrome has given us immense power by allowing us to run it headless. A programmable browser lends us a lot of control to automate our UI tests now.
Puppeteer is a Node library which gives a higher level API to control headless Chrome. We can automate tasks like opening a browser like opening a URL, clicking a button etc. using Puppeteer. As a result, it has taken UI testing to a whole new level.
The purpose of this article is to simplify the process of writing tests using Puppeteer.
Setting up a node app with Puppeteer
Let us create a folder named tests in your work directory.
mkdir tests
Add a file package.json to tests folder.
vim package.json
And add the code below to your package.json
{
"name": "tests",
"version": "0.0.0",
"private": true,
"scripts": {
"start": "node test.js"
},
"dependencies": {
}
}
To install puppeteer, run the code below. This may need some time as it will download the Chromium which is around 100mb in size.
npm install --save puppeteer
or "yarn add puppeteer"
Once you have added Puppeteer in your directory, you are ready to write tests for any website.
How to write my first test
Create a file named myfirsttest.js
vim myfirsttest.js
Add the code below in your file myfirsttest.js. These are the functions to open and close the browser. Basically, before starting any of your test cases you are supposed to start a browser and at the end, you are supposed to close the browser. This can be achieved by these two functions.
const puppeteer = require('puppeteer');
async function startBrowser() {
const browser = await puppeteer.launch();
const page = await browser.newPage();
return {browser, page};
}
async function closeBrowser(browser) {
return browser.close();
}
For writing a test case, add a new function playTest to your file myfirsttest.js. Start your test by opening the browser and then open a page with the URL on which you want to run your tests. To keep things simple, I have added only a snapshot in the article and close the browser at the end of the test. You can write whatever else you would like to do with the page in here.
async function playTest(url) {
const {browser, page} = await startBrowser();
await page.goto(url);
await page.screenshot({path: 'screenshot.png'});
await closeBrowser(browser);
}
For running the test, add these lines to your file:
(async () => {
await playTest("https://browsee.io/");
process.exit(1);
})();
Once you are ready with your file, run it on the command line by:
node myfirsttest.js
This will generate a snapshot screenshot.png in the same folder that is essentially the snapshot of Browsee home page.
As you can see, I have not discussed async/await in details here. You can read about it in detail here. If you are finding it difficult to understand the concept, I would say just take some time and consider this as a format of language. As in a browser, you wait for things to load before clicking or scrolling or typing, you need to put a simple await before any step that will take time. For example, open a browser, go to URL, taking a screenshot or closing the browser. Just put await on any steps like that and follow the language, you should be easily able to test your website with Puppeteer!
If you would like to know when your users face bugs or errors, please check out Browsee. It is user analytics platform where you can see how users actually used your site and learn from it.
How much is a great User Experience worth to you?
Browsee helps you understand your user's behaviour on your site. It's the next best thing to talking to them.
